5.5 Assembly
Assembler, assembly, ASM and binary hacking are some of the synonyms for this and although technically they have slightly different meanings for the most part if you say one and you have enough context nobody will really say anything although binary hacking is perhaps accurate but not really advisable.
Although all the other techniques mentioned in this guide have examples of highly specialised knowledge at the heart of them in many ways ASM hacking has the highest barrier to entry and similarly is the most far reaching for much like using a hex editor to edit everything it might not be advisable but anything can be edited using ASM techniques. So as not to beat around the bush learning ASM will mean you are learning one of the hardest, most specific (write something in Java on one system and it will take minimal or no real work to have it running on something else that has Java but write something in ASM for the GBA and you will probably have a very hard time getting it to run even on the DS) and most involved types of coding and if you ignore some of the Esoteric programming languages probably the hardest. This however is not to say that is not worth learning at least some of the basics as it will help with other areas (as is a common theme in this document knowing how the hardware works will allow you to push things to the limit without going over or being able to crawl back if you do) and more importantly some of the techniques can be done without much appreciation for what they are actually doing behind the scenes; you might not have a deep appreciation for the nuances of the memory and memory reading systems for a console but anybody can set a breakpoint and wait for the given instruction to write to a given area and see where it reads from.
It should also be said although assembly programming is extremely powerful it is still a programming language so merely knowing it will not turn you into a computer scientist even if it does often make them easier to learn at first so continue to learn other concepts; if it looks like those that know assembly know the rest they probably do but they almost certainly learned it along the way.
The obvious question of the reason we care to do all this is everything is eventually rendered in assembly (even if it is at runtime as is the case with some the higher level languages) and if everything is rendered in it everything is understandable through the filter of it and everything is editable with it.
Although this section aims to be a reasonably complete introduction to assembly you are also encouraged to have a full hardware specification like gbatek available as it will contain things this omits and be more useful as a reference.
5.5.1 ARM
The GBA uses an ARM7TDMI processor where the DS has an ARM9 processor (specifically a ARM946E-S) and an ARM7 which in practice is a higher clocked version of the GBA processor22. The instruction set they run is rather confusingly known as ARMv5 but not so many people refer to this with ARM9 and ARM7 being the usual things people referred to.
They both have two modes of operation with the main one being ARM and the secondary one being THUMB; although the term secondary is used and it is in many ways the weaker of the two modes games can and quite frequently do spend large periods or even most of their runtime in THUMB mode.
It is no bad thing if you are used to the likes of the X86 with nested registers and the many many quirks let alone having to optimise things for it but the processors themselves are fairly basic as you would expect from a RISC architecture with the only real omission being a lack of a divide instruction. On the subject of dividing the BIOS will be covered later in more depth but it does provide a divide instruction although it could be faster and some games will still implement log tables and similar methods (the GBA BIOS goes a step further and provides square root and arc tangent) and the DS ARM9 also has a coprocessor of sorts for maths that uses IO to function and supports divide and square root there.
A selection of links dealing with the various processors
Arm architecture (general concepts)
Quirky’s things you never wanted to know about assembly
imrannazar.com ARM7 and 9 opcode map
Smallest ds file (not so much assembly but worth reading)
crackerscrap.com (click documentation) (covers several example assembly techniques).
microcross.com GNU ARM assembly quick reference A small reference document to some of the directives supported.
Silicon errata Programs can have bugs, chips can have bugs and as processors are increasingly a combination of the two they can definitely have bugs to say nothing of the architecture as a whole; as various points in this document have spent time covering the GBA and DS augment their processors with fixed onboard hardware also capable of processing at some level. Now where there are certainly undocumented features at time of writing there is not much known about any silicon errata for the GBA or DS compared to the likes of x86 processors although GBAtek covers several errors and odd design choices (remember ARM stuff tends to be custom/application specific where the likes of the x86/x64 processors are largely standard and almost certainly are until you get to very high levels of science, industry or enterprise). This is further abstracted away from programmers by most compilers, in some cases assemblers and toolchains being updated to work around them which could trouble ROM hackers as it will not tend to filter down to them. In practice it is more likely to be the hacker that is at fault or the emulator that does not handle a specific condition (remember in practice emulation is usually an attempt to make an approximation of a system in code that runs at a reasonable speed) and maybe an assembler issue; games consoles are often said to use off the shelf CPUs but they do occasionally have some tweaks as indeed was seen on the “Z80” the GB/GBC used.
On a slightly higher level if you read hardware documents for the GBA and DS you will probably come across prohibited modes and a few mentions of hardware bugs which usually are related to each other and are about as close as you will get to seeing something like silicon errata.
5.5.2 GBA Assembly specifics
The following section has details on the GBA hardware itself and key things to know and some basic techniques to employ that make assembly hacking easier.
GBA memory The GBA has several memory sections although most are usually concerned with the VRAM, the OAM, the WRAM, some IO and the cart itself.
The cart itself is usually read from the 08000000-09FFFFFF region (a full 32 megabytes) hence most pointers on the GBA being assumed to be either to the WRAM or the 08XXXXXX region (most ROM images are 16 megabytes or less so the 09 section and equivalents are rarely seen23 ). There are however two other waitstate locations also running for 1FFFFFF known as WS1 and WS2 at 0A000000 and 0C000000 and by default they are slower/have lower priority than the WS0 location. By default was the key word in the previous sentence as 4000204 hex otherwise known as the WAITCNT register can change this.
Location | Size | Description |
00000000 | 3FFF | BIOS |
02000000 | 3FFFF | WRAM |
03000000 | 7FFF | WRAM (on chip) |
04000000 | 3FE | I/O locations/registers |
05000000 | 3FF | Palette RAM |
06000000 | 17FFF | VRAM |
07000000 | 3FF | OAM section |
08000000 | 1FFFFFF | Cartridge location |
0A and 0C | 1FFFFFF | Cartridge location WS1 and WS2 |
0E000000 | Varies | SRAM location |
Basic overview At boot the binary is loaded and run (covered below) and runs from the cart itself with everything usually24 being streamed to the memory as necessary via a combination of DMA and SWI calls that effect a memory transfer by the way they work. Owing to the somewhat low resources things are fairly tightly managed on the GBA and things in memory tend to be there for a reason.
DMA GBAtek covers them is great detail but there are four channels numbered 0 to 3 and have an ascending priority (the others are paused until the higher priority channels are done). Each has an independent control register (which can disable the channel it is responsible for) and three write only registers to control source (DMA0 can do only internal memory and will not read higher than 7FFFFFF but the others can do for carts as well), length of read (word count) and destination. The control register does more than just control it and can be set to start immediately, at a vblank, at a hblank (this is aimed at DMA0) and special cases which depend on the channel.
GBA Binary The GBA protocol known as multiboot is not really going to be covered here as most hackers do not tend to deal with it mainly as most commercial ROM images do not fit in it, not to mention it is covered in hardware documents.
The GBA binary (as in actual executable code) is mixed in with the ROM image itself but it is easily found for the vast majority of games. Trainers for ROM images (be they the ones Scene groups put on games or ones used for trainers) will tend to subvert this to run before the game.
After the BIOS loads and the Nintendo logo is checked the first thing the game looks at is the very first address in the ROM (08000000) which is the start of the header and typically contains a jump instruction.
This jump usually jumps to the end of the header where things start getting set up, very useful for the program to come but not really what you are really looking for. This part is found a few lines down when the resulting read is next pointed at a value in the 08XXXXXX range and that is the start of the GBA binary that actually makes the game does what it will.
Some games like Phantasy star collection will have multiple executables in the game (after an initial load menu) but this is rare and for these you will have to use an emulator like VBA-SDL-h or no$gba debug or manually run through the assembly in long form to find it. Although technically possible no full commercial game, homebrew is a different matter, at time of writing has been observed to copy executable code to WRAM and operate there like the DS does with its binaries. This is presumably owing to the GBA cart being reasonably fast, low latency, having a prefetch command, the WRAM not being that large (288 kilobytes or 256 if you only use the system WRAM) and the GBA cart being mapped to memory. Indeed this is expected by many ROMs which will crash if you use slower memory which is why the simpler (and usually better) GBA flash carts use PSRAM or NOR as opposed to the cheaper and easier to work with NAND flash memory. There are a couple of games that might run the odd decompressed function from WRAM though, multiboot can make use of it and GBAtek notes that many save functions need to be executed from instructions in the WRAM.
Screenshot of VBA’s dissassembler showing how to find the binary The image displayed below shows what the basic disassembly looks like with a few extras to help you out. The data bounded by the yellow box (which has been shrunk for the purposes of readability) is what the disassembler makes of the header and is a nice reminder that disassemblers, for all they might allow, are just like hex editor ASCII windows in that any good they display is luck, good standards (which do not apply so much here) or the end user guiding it to display something useful. The general process in words is the start of the ROM houses a jump, usually to the end of the header but otherwise slightly later in the ROM, which is the start of the real binary but most of what immediately follows that is basic setup so the first thing to deal with something in the GBA binary region (that is to say not the stuff setting the stack pointer) is where the meat of the binary is.
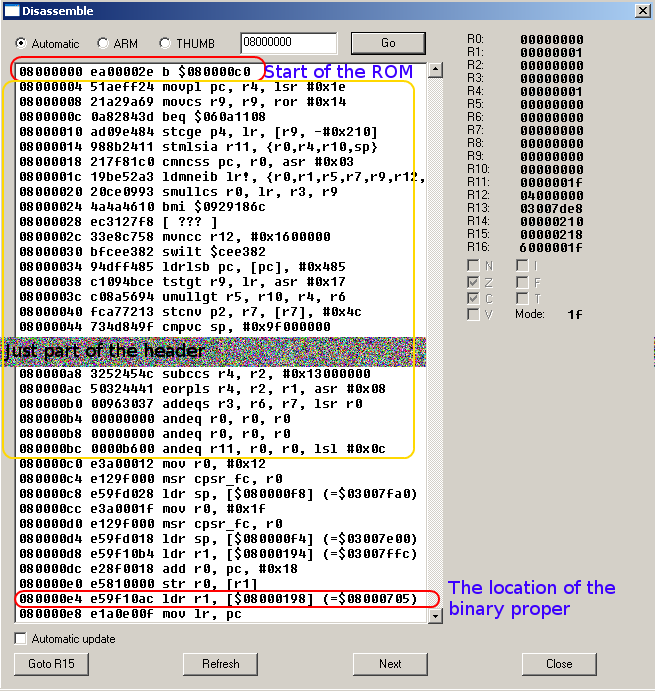
PIC
Tracing Tracing is the process of finding out where something started out at in the ROM and how it got to where it is which is usually just a read of some form but there is occasionally compression in the way meaning you will have to find where the compressed data got into memory from and then repeat the process with that.
Locating graphics with VBA-SDL-Hby Labmaster provides a great introduction to the subject and there are a few worked examples to come in part III.
The VBA-SDL-h website and help files also include a listing but a breakdown of commands and options is still useful. Some debugging tools (especially those on the DS) are less featured and some programs like IDA have more features in some cases but the standard set is
- Breakpoints
- Run until
- Read memory and registers
- Change memory and registers
- Search memory
- Maths (VBA-SDL-h supports boolean logic and conventional maths)
- Variables
- Logging
The VBA-sdl-h debugging side of things is a command line affair (press f11 when the game is running to access the debug features and when done enter a command of c and it will continue) and much of it is fairly obvious but a quick discussion of what goes is useful.
Breakpoints - these come in various types with break on read from an address and break on write to address (and a window a window of a given length afterwards if you want) being the most useful when tracking down files and doing basic reverse engineering. Break on either a thumb or arm instruction at a given address is available and quite useful too.
Run until - technically another type of breakpoint but here you can set a point in the ROM and when that address is read as part of an instruction the game will stop. This is usually one of the first commands a debugging emulator will gain (certainly the DS emulator iDeaS which lacks a few functions VBA-SDL-h has can do this).
Memory - writing to memory has been seen a handful of times already and when dealing with assembly level stuff it is even more useful.
Read memory and registers. With memory and registers tending to the core of the (note this tends to mean CPU registers rather than IO registers but those are evaluated easily enough). Likewise search options are available for both hexadecimal and ASCII
Evaluate memory with boolean/maths done. As has been seen several times values can mean a lot of things and not be immediately apparent but a simple bit of boolean logic or maths will turn it into plain text (think back to compression where three was added to the length value) and VBA-SDL-h then has the ability to do a bit of basic maths on the results of a read.
Variables/expressions are supported in VBA-sdl-h and are not really typecast (the closest it comes is anything with a $, 0x or leading 0 is evaluated as hex and anything else as decimal) so you can have a number as a variable for use in maths or you can also have the number be a memory address you need memorised or to be able to referred to with a nicer name than a bunch of numbers. Registers of the CPU also have their conventional shorthands of R0 through R15 although PC, LR and SP are available as well.
Logging has been seen in the past (SWI logs being used to assist with compressed ROM images) but the idea here is rather than stopping every few seconds (if you have ever used a whitelist program or sandboxing/confirm big changes programs it is a similar feeling after a few breakpoints have been set) everything is logged for later examination.
5.5.3 DS Assembly specifics
Much like the GBA section above this section contains information on the DS hardware, general modes of operation and some techniques you can use to help get the most out of assembly.
DS Binaries Types of binary
There are three main locations you will find DS binaries.
- The standard binaries and overlays (arm9.bin, arm7.bin and overlay_????.bin where ? is a decimal number).
- Binaries and overlays contained within download play files (utility.bin, especially if found in dwc directories)
- SRL files (usually a developer/debugging leftover).
The standard binaries are the arm9.bin and arm7.bin files you will find in every DS ROM. Overlays are not restricted to the ARM9 but in practice as the ARM7 is something of a static binary for commercial games used for basic tasks ARM9 tends to be the only processor to have them; in practice there usually less than ten but some ROM images go up to around one hundred although some go further and break the one thousand mark.
As for what overlays actually are there are occasions where you might want to extend the functionality of your code but you do not want to have to take up a chunk of valuable memory at all times despite the game only needing it every 3 hours or so. This is a common occurrence throughout computing and the way the DS chose to handle it was too look back in time to overlays which amount to having small fragments of code you can load into a given memory location and then run before releasing them and swapping in another fragment. It is quite possible to have overlays with different intended running locations and having multiple overlays run at the same time. Most header viewers like NDSTS will tell you where the ARM9 is found in the ROM, located in the RAM (it can vary between games) and has the initial point of execution and although you can read the data out yourself you might have to go to a program like Crystaltile2 to get information on the overlays.
DS memory The DS avoids mapping some things to the main memory bus with full read/write access (some parts of the firmware, the cartridge itself, the 3d RAM and some aspects of the touch screen to name a few big ones) which troubles some things but a lot of it is still mapped to the main memory bus and it is definitely still a useful concept. Equally the two processors have slightly different maps although there is a lot of overlap.
ARM9 (start-end) | size | Description |
00000000-0AFFFFFF | - | Total memory mapping* |
*Aside from ARM9 BIOS at FFFF0000 for 32 KB. | ||
00000000 | 32KB | Instruction TCM |
01?????? | 32KB | TCM (tightly coupled memory) |
GBAtek on the TCM. | ||
02000000-2400000 | 4MB | Main ram section |
03000000 | Shared WRAM | |
04000000 | ARM9 IO | |
05000000 | Palettes | |
06000000 | Video RAM (VRAM) | |
06000000h | 512kb | VRAM - BG for Engine A |
06200000h | 128kb | VRAM - BG for Engine B |
06400000h | 256kb | VRAM - OBJ for Engine A |
06600000h | 128kb | VRAM - OBJ for Engine B |
07000000 | OAM | |
GBAtek has more on some of the VRAM quirks (see LCDC) | ||
08000000-09FFFFFF | 32MB | GBA ROM (no mirrors in DS mode) |
0A000000 | GBA save RAM |
ARM7 | size | Description |
00000000h | ARM7-BIOS (16KB) | |
02000000h | 4MB | Main Memory (shared with DS) |
03000000h | 0-32KB | Shared WRAM (0,16 or 32) |
03800000h | ARM7-WRAM (64KB) | |
04000000h | ARM7 I/O | |
06000000h | 256K max | VRAM allocated as Work RAM to ARM7 () |
08000000- 09FFFFFF | 32MB | GBA Slot ROM |
0A000000h | 64 | GBA Slot RAM |
On shared WRAM The DS allows a small section of ram to be shared/split between the ARM9 and ARM7 and it is mirrored repeatedly although in this case 37F8000 hex is the interesting one which allows for (and a couple of programs have been seen to use) a single 96 kilobyte block for the ARM7 as it has WRAM right after it. WRAMCNT controls the allocation although only the ARM9 can do anything about it.
TCM On the face of it most people would assume the TCM is a type of CPU memory cache and although it could be used as such a feature in practice it is a small memory block that can continue to operate during DMA transfer (assuming all it needs is inside it) although DMA can not access it. GBAtek has more.
DS IO section 04000000 for both the ARM9 and ARM7 house IO for the DS which includes values of controller input, DMA, screen handling, sound as well as the locations to used for the maths coprocessor/functions (a signed 32 or 64 bit division function clocking around 18 clocks for all 32 bits or 34 clocks otherwise although it has a finished flag and a 64 bit square root function lasting 13 clocks with a 32 bit output).
GBAtek naturally has a complete listing and covers their contents and usage in the relevant sections and a lot of it was already covered when graphics and sound were covered.
On DS memory usage With most DS files using file level pointers there can be the impression you are granted as much memory as the pointers will afford and indeed many games will within reason be able to manage it to the point you are more likely to run into problems with screen positioning. Some games however do load say the entire script or file into RAM and use it from there meaning you either get to limit your text (or more likely if it is a Japanese game do an 8 bit text encoding conversion), code in a streaming ability or try something more exotic like trying to store things on the GBA cart or abusing the file level pointers to point somewhere in RAM and injecting it there somehow (remember values are probably calculated from pointers in the file to guide things once it is found in RAM so you could get something working there by using a far larger number than it expects).
VRAM on the other hand you will frequently run into problems when manipulating and developers will often have pushed it to the limit here.
On cart access The cart access is controlled through IO (usually the B7 command to 40001A8) and that is a good thing to watch as games are not necessarily restricted to a single read function (indeed the Bink video format from Rad game tools boasts the ability to handle file reads in library in the Bink video format sales patter) so watching a single function might not net you what you want. This being said it is by no means a bad idea to watch a single read function if you find one for a while to get a handle on things and owing to the nature of the DS filesystem it quite often can boil down to a small bunch of functions attempting to figure out enough pointers to find the given section in the ROM itself (think in SDAT there might be a track within an SSEQ which is within a section which will have a point to that section as part of the SDAT file which will have a pointer in the FAT section of the DS ROM which itself will have a pointer listed in the header).
**crystaltile2/no\(gba ASM markup aka NEF** Nintendo developed a kind of markup format for their debuggers to use which no\)gba (at the time the premier debugging grade emulator for the DS) and, eventually, crystaltile2 gained support for. no$gba also supports a format it calls sym which at times almost feels like an extension of nef.
The format allows you to assign names to memory locations (be they variables, names for IO or locations of functions/loops), declare sections to be data (8,16 or 32 bits per entry for a given number of bytes), declare sections to be just THUMB or ARM mode code and write comments and such similar to a development assembly environment for although assembly is about getting right down to the bare metal it is trivial and of no concern to the resulting speed of a program to have a nice name for a memory location defined when programming, used in the programming and replaced at time of assembly.
SRL/download play SRL files are binaries output by debugging tools. For the most part if they are seen in ROM images they are usually a developer left extra, sometimes though they are there as or in download play components as the main binary. Download play ROMs can and frequently do use a regular style DS binary instead (usually called utility.bin), do note they usually use a lot of compression and just about everything in a utility.bin file will be compressed.
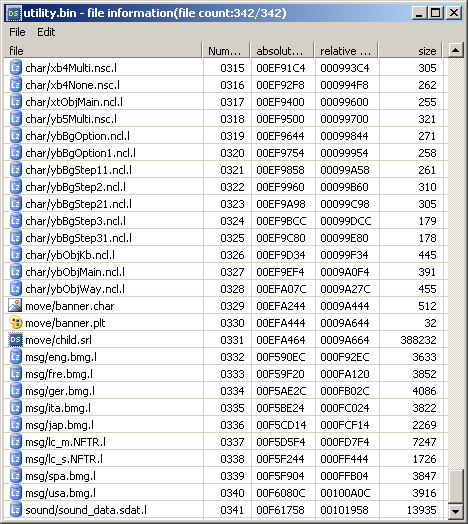
PIC
Binary compression It was mentioned back when compression was covered but it should be noted the DS binaries and overlays can be compressed, some Scene groups even compressed them if the original ROM had not compressed it. The standard compression method used in DS binaries is a type of LZ compression sometimes known as BLZ (backwards LZ) or more often DS binary compression. Tools like crystaltile2 handle the decompression (although with crystaltile2 you are advised to rip the file normally and decompress with one of the following tools if you plan to reinsert it, crystaltile2 has also been seen to say there is compression where there is none) as will tools like Cue’s compression tools and DSdecmp. Equally the binary has to be decompressed to run so you could always snatch it out of RAM, which was actually something many did do before the format had tools made for it.
No binary encryption has been observed, though some called the binary compression a type of encryption at times and obfuscation is seen on many occasions. Equally there are a few scripting languages available for DS programmers so there may be some amount of just in time compilation and similar things.
5.5.4 The GBA and DS compared
The following section will compare and contrast the GBA and DS as well as elaborate further on the functions of each.
The GBA, unlike the GB/GBC predecessor, does not have BIOS level sine tables (remember sine can be used to generate cosine and tangent values) but the DS ARM7 does, even if developers will still tend to implement tables or things at feature level, as SWI 1A.
Other than speed the differences between the processors are minimal as well with the ARM9 (ARMv5) have an instruction to count leading zeros, another branch code and the ability to set a breakpoint; the different processors do have access to a different BIOS (and so a few different commands) and different things (I/O and hardware) within the system though. This being said although the DS has two processors as mentioned a few times the ARM9 is the thing that does most of it for the purposes of commercial games (homebrew is a different matter and there are several examples of things using the ARM7 extensively) and the ARM7 can for the most part be considered a slightly higher powered IO and/or premade functions system. The speed bump has resulted in an increased use of compression but most of it is fairly standard and with a filesystem it is not hard to work with.
Regarding SWI/BIOS functions most of the GBA sound functions are gone on the DS and a few have different mappings but generally speaking they are the same.
IO is much the same save for the extra buttons, touchscreen and real time clock (which was an optional on cart feature on the GBA) but the real change is where the GBA BIOS functions for a lot of maths the DS has a maths coprocessor of sorts.
On the face of it the DS has a lot more main memory than the GBA (288 KBytes for the GBA and just over 4 megabytes by the time all is said and done for the DS) but owing to the cart not being memory mapped this is not quite as big a jump as it might seem when it comes to actually running things as the ARM9 binary has to appear in memory and most of the time files will have to be copied from the cart rather than accessed directly as it was on the GBA. DS cart access is also somewhat slower than the GBA and although a few games have come off worse for it as far as most day to day use is concerned it is more than workable.
Interrupts are largely the same save for a few extras on the DS to handle the newer hardware, they are however restricted by processor with the SPI (used for DS saves and on card bonus functions like the pokewalker for some of the later pokemon games) and wifi being taken care of by the ARM7.
DMA is much the same on the face of it although both processors can get in on it effectively doubling the DMA channels. The ARM7 is much the same as the GBA but the ARM9 one is expanded and has a few more modes to handle new sources of data and work with the 3d as well as losing some of the restrictions so it now has full memory access for all channels.
VRAM and graphics. Even with two screens taken into account the DS has quite a bit more VRAM than the GBA although it is still not enough that you can never run out/sections will be redundant however much like the general system RAM the difference is not apparent as streaming directly from other parts of the memory is quite possible; better yet other than the final rendered image from the 3d engine nothing really touches the 2d VRAM memory that is not graphics related. The different engines in the DS are not the same and can take a bit of time to figure out to say nothing of the interplay between them but for the most part they are fairly logical.
The 3d engine which was new to the DS is for the most part not memory mapped/readable in memory although the registers to control a lot of it are visible in ram in an emulator even if they are write only on original hardware; the rendering and geometry functions are effectively given separate memory areas and much like other parts of the system 3d is somewhat IO driven. It is fairly weak as far as 3d hardware goes (as a testament to that unlike most 3d engines fixed point operations are the order of the day) leading a fair few counts of precalculation and tricks on the part of the developer to get as much as possible out of it although various levels of texturing, lighting, fog and shadows are available.
5.5.5 On controls
There may well come a time where you wish to change the controls of a game and people typically approach this from three main directions
- Cheat/miss activator
- Memory tweaking
- Game editing
The activator is the favoured method of most cheat makers (although do be careful not to confuse it with some onboard button activators a cheat engine might afford you) with a great example being the Trauma Center “miss to refill health”; here certain points in the game required the patient to be at a lower health to trigger a further event so the infinite health cheat had to become a refill health when a “miss” happened.
Memory tweaking is probably the most common and works as the state of the buttons on the GBA and DS are mapped to memory and so can be accessed; in practice though many games will copy this value and operate upon that instead which has the added bonus of lessening the effects of a button suffering from bounce failure (most buttons are just switches and might display open and closes several times over the course of one press; if you have ever had a mouse start to double click after being used for a while it was probably an example of it).
The GBA and DS at 4000130 hex for 16 bits has the standard GBA buttons (no X and Y) for both consoles.
The DS has an additional 16 bits at 4000136 hex for the X,Y, screen closed, “debug”, and whether the touchscreen is being pressed (no position data for this as that is covered elsewhere).
It gets slightly more complex as there are interrupts in there) with on bit for each of the various buttons.
Game editing is what most think of when they first hear of the term and here there will be an interrupt or check set to read the control memory (be it the mapped section or the copied version) and act accordingly. Here the functionality of the game that does similar will be reworked. It should be noted that if a game has the option to remap controls, even between a small selection of premade maps, then it may be better to look at what goes with that abstraction.
DS Touch screen The DS has a touch screen in addition to the buttons. Various hacks got done but as Nintendo pushed the touch screen quite hard on developers (their first party offerings even more so) quite a few games ended up with touch screen controls even though the games themselves were arguably better suited to button controls. Step in ROM hacking and a bunch of games had their touch screen controls remapped to buttons with two of the most notable being those in the Zelda series and Starfox, Crackerscrap.com guide to touch screen to controls hacking has a nice worked example of various methods to this. It should also be noted that the DS touch screen had something resembling a conventional button to simply say the screen was being pressed in addition to all the options afforded by actual movement.
Looking back to the level editor in the N+ level editing section where 020E0840 was a touch screen driven location of the selection box it might have done to instead select that as the intended destination of a controller hack.
Extra peripherals The GBA and DS carts both have GPIO (general purpose IO) options built into them which have been used at various points to do various things. On the DS this was mostly the GBA slot used for rumble, the taito game pad and the guitar grip for guitar hero. Indeed the cheats made to allow buttons to be used instead of the guitar grip saw the memory section that the guitar pad button states were copied/debounced to be fiddled with.
Other hacks here included support for the the Taito paddle controller (originally intended for the Japanese version of Arkanoid) to other games like Mario Kart DS.
5.5.6 Hooking
Some further discussion is available in the following section (see destructive vs non destructive assembly editing). However even if you can understand the disassembled code well enough to recreate the original source code then as far as a lot of hacking is concerned that is only half the battle as you will probably also want to edit and run the code. The process of interrupting the ROM and changing what it does/running your own code requires you first to get a point where you can tear it away from the original code, or, to use the proper term, hook the code. There are three main classes of this
- Instruction editing
- Subverting functions
- External hooking
Instruction editing was mentioned back in cheat making where if you found the instruction that removes a life and NOPed it or changed it to an add or something this would be it. It tends to get very complex to do this for anything other than a basic hack unless you jump somewhere else, a practice which is usually reserved for subverting functions.
The classic case of subverting functions would be in the case of adding a variable width font to a game and where the game would usually calculate what it needs to do you change the games default font handling code to your function you buried somewhere else.
External hooking is probably the only method that actually warrants the term hooking and has more in common with things like cheating devices. On the DS at least there is the ARM7 which has comparatively little functionality in commercial games but more or less full access to the memory so here you can set an interrupt to run at some arbitrary time and do what needs to be done. Similar things were done for the generic soft reset and sleep mode patches on the GBA which set interrupts to run if a given button combination was pressed.
5.5.7 GBA cart as extra memory for DS hacks
As far as general ROM hacking is concerned this is still largely theoretical but ask any ROM hacker if they would like the system to have an extra 32 megabytes of memory that is low latency, high speed and addressable directly in memory the answer is likely to be yes but it will never happen. The DS and DS lite however feature the GBA slot which is 32 megabytes and all the above although a select few games will use the header section and maybe save section to gain extras, homebrew might use it and the web browser will also try to use it (in these last two cases case it is actually read/write though) it still leaves a very large amount of space available. Here the pointer in RAM that starts 02 could in theory be changed to start 08 (or 09 for the upper 16 megabytes) and as DMA restrictions were lifted on the DS anything that might have to go from the 02XXXXXX memory section to somewhere else should not be troubled.
Some people attempted to clock the various ARM7 in GBA mode in various consoles (GBA, SP, GBM, DS and DS lite) and they are usually just a few tenths of MHz off between each other.↩︎
Of course with no bank switching to speak of that usually means there is 16 megabytes or of free space quite able to be used just by pointing there which many hacks have done. It should be noted that several of the dominant GBA compatible flash cards and some of the embedded emulators are not so fond of files larger than 16 megabytes although the former can still handle them. To this end if you can repoint and keep it under 16 megabytes do so and if you have an 8 megabyte ROM then use the remaining 8 megabytes first.↩︎
Various programmers in both the homebrew and commercial side of things have been seen to attempt to do things to the memory using standard CPU memory write/read functions and although they are not unbearably slow they are far from suggested practice.↩︎